I can use KoolControls in a standalone project without any problems, see code below:
<?php
/*
* Please put this file in the same folder with KoolControls folder
* or you may modify path of require and scriptFolder to refer correctly
* to koolgrid.php and its folder.
*/
include "KoolControls/KoolGrid/koolgrid.php";
include "KoolControls/KoolAjax/koolajax.php";
include "KoolControls/KoolGrid/ext/datasources/PDODataSource.php";
$koolajax->scriptFolder = "KoolControls/KoolAjax";
$DB_HOST = 'localhost'; //Host name<br>
$DB_USER = 'sakila'; //Host Username<br>
$DB_PASS = 'caroline'; //Host Password<br>
$DB_NAME = 'sakila'; //Database name<br><br>
$ds = new PDODataSource(new PDO ("mysql:host=$DB_HOST; dbname=$DB_NAME;", $DB_USER, $DB_PASS, array(PDO::MYSQL_ATTR_INIT_COMMAND => "SET NAMES utf8"))); // PDO Connection
$ds->SelectCommand = "select actor_id, first_name, last_name from actor";
$grid = new KoolGrid("grid");
$grid->scriptFolder = "KoolControls/KoolGrid";
$grid->DataSource = $ds;
$grid->styleFolder = "default";
$grid->Width = "655px";
$grid->Height = "300px";
$grid->PageSize = 25;
$grid->AjaxEnabled = true;
$grid->KeepRowStateOnRefresh = true;
$grid->AllowScrolling = true;
$column = new GridBoundColumn();
$column->DataField = "first_name";
$column->HeaderText = "Vorname";
$column->Width = "45%";
$grid->MasterTable->AddColumn($column);
$column = new GridBoundColumn();
$column->DataField = "last_name";
$column->HeaderText = "Nachname";
$column->Width = "45%";
$grid->MasterTable->AddColumn($column);
$column = new GridBoundColumn();
$column->DataField = "actor_id";
$column->HeaderText = "ID";
$column->Width = "10%";
$grid->MasterTable->AddColumn($column);
$grid->MasterTable->AllowHovering = true;
$grid->MasterTable->VirtualScrolling = true;
$grid->MasterTable->AllowSelecting = true;
$grid->MasterTable->AutoGenerateColumns = false;
$grid->MasterTable->AutoGenerateDeleteColumn = false;
$grid->MasterTable->AutoGenerateExpandColumn = false;
$grid->MasterTable->Width = "655px";
$grid->MasterTable->RowAlternative = true;
$grid->MasterTable->Pager = new GridPrevNextAndNumericPager();
$grid->Process();
?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title>KoolGrid</title>
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
</head>
<body>
<form id="form1" method="post"> <!-- You should render the grid inside a form tag, in case $grid->AjaxEnabled = false the grid needs a form to operate. -->
<?php echo $koolajax->Render();?>
<?php echo $grid->Render();?>
</form>
</body>
</html>
This gives me a nice grid with default style, virtual scrolling, ajax and pager:
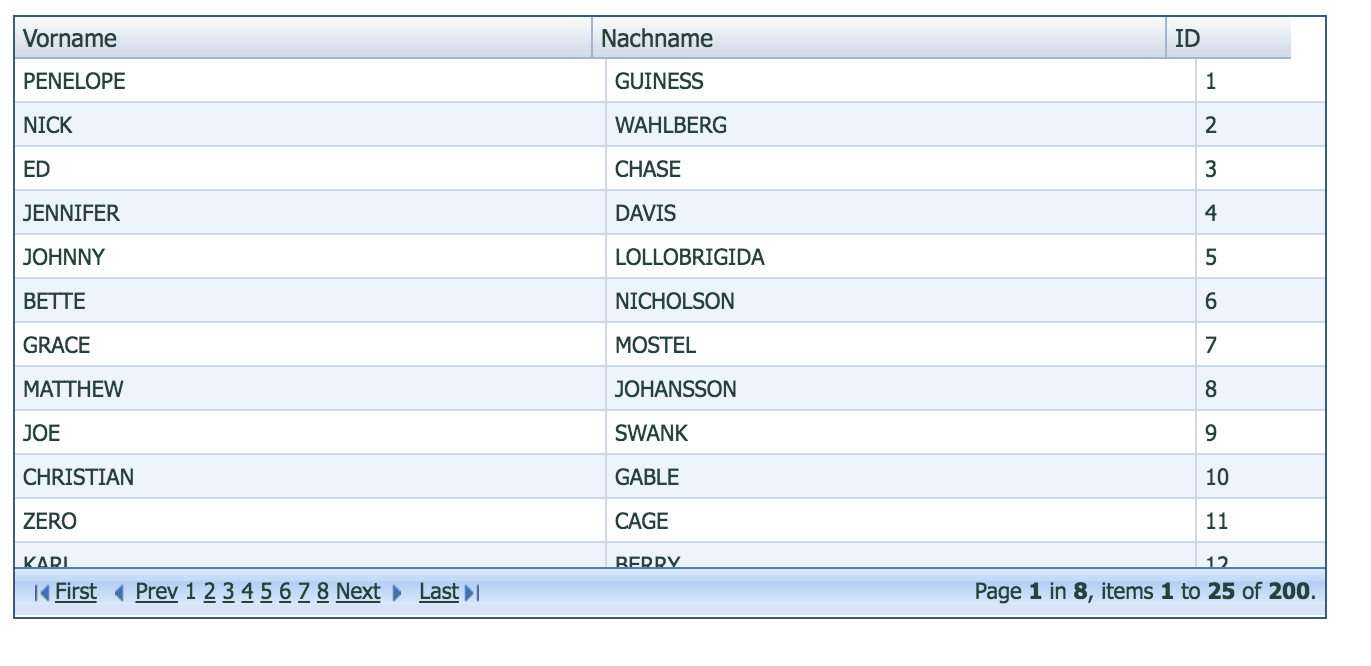
However, if I put the very same code in a view file in CodeIgniter and the KoolControls folder in the same folder, I am getting a grid without style, virtualscrolling and pager which does not work:
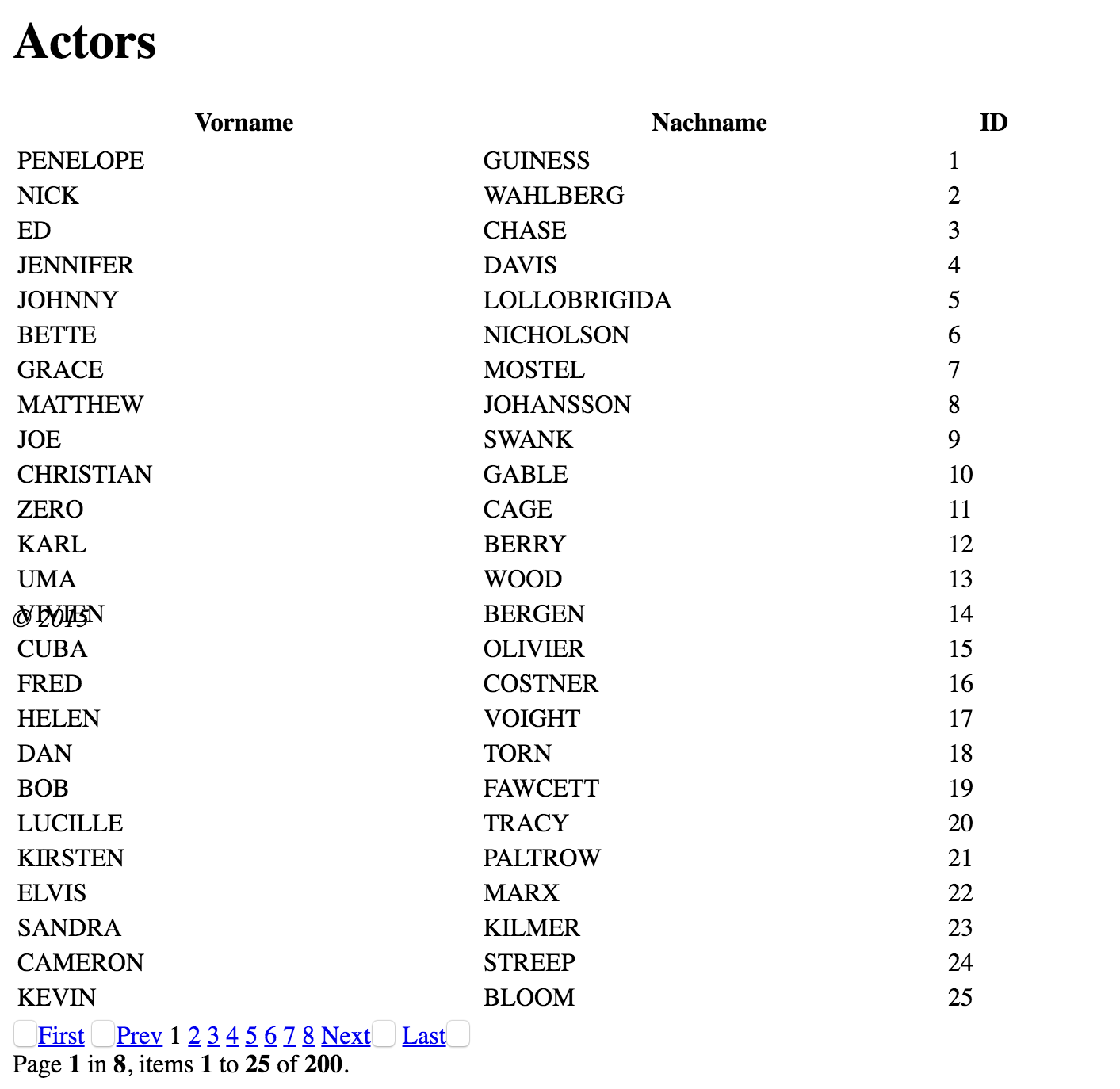
Here is the directory structure:
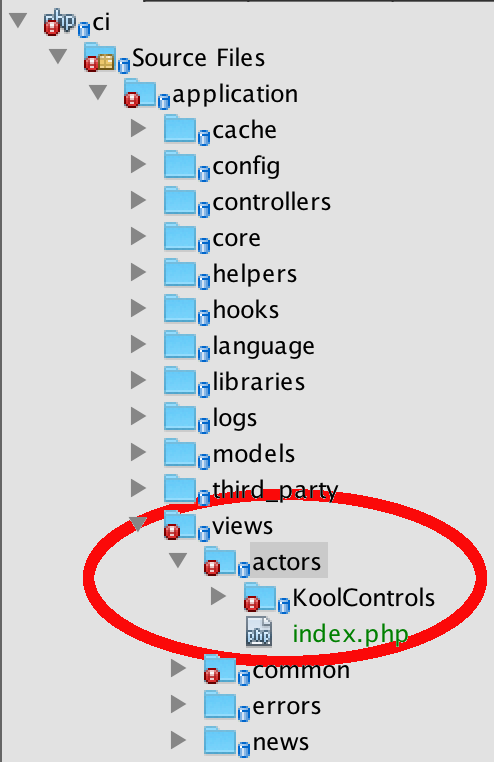
I dont know why KoolGrid works nicely as standalone, and not inside CodeIgniter.
Note, ideally, the KoolControls folder should not reside in the a particular view folder of CodeIgniter, but directly in the 'view' folder so it can be used from all views, but i could not make this work either.
KoolControls are real good components, but if they don't play nicely together with PHP Frameworks, they are worthless. No one will build complex PHP applications without a framework.
I hope you guys at KoolPHP will fix this soon.